– In this post we are going to learn, create simple chat in asp.net using C# language.
STEP 1 – First Create asp.net web application and Create Three web form named Home.aspx, Chatting.aspx and Msg.aspx.
STEP 2 – Design Home.aspx form like below :
Here is the HTML code for Home.aspx
<body>
<form id=”form1″ runat=”server”>
<div>
<table style=”z-index: 100; left: 344px; position: absolute; top: 160px”>
<tr>
<td colspan=”3″ style=”text-align: center”>
<strong><span style=”font-size: 16pt; color: #0000cc”>Chat Application Login Area</span></strong>
<br />
<br />
</td>
</tr>
<tr>
<td style=”width: 100px; text-align: right;”>
<strong>
Name : </strong>
</td>
<td style=”width: 100px”>
<asp:TextBox ID=”txtname” runat=”server”></asp:TextBox></td>
<td style=”width: 100px”>
</td>
</tr>
<tr>
<td style=”width: 100px”>
</td>
<td style=”width: 100px”>
<asp:Button ID=”btnlogin” runat=”server” OnClick=”btnlogin_Click” Text=”Login” /></td>
<td style=”width: 100px”>
</td>
</tr>
</table></div>
</form>
</body>
The Output of Home.aspx
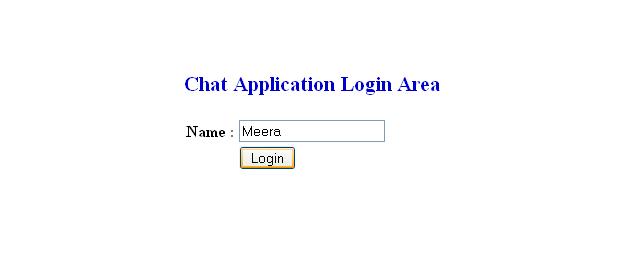
STEP 3 – Now, Design Msg.aspx page like :
<html xmlns=”http://www.w3.org/1999/xhtml” >
<head runat=”server”>
<title>Chat Message</title>
<meta http-equiv=”REFRESH” content=”2″>
</head>
<body>
<form id=”form1″ runat=”server”>
<div>
<asp:TextBox ID=”txtmsg” runat=”server” Height=”290px” ReadOnly=”True” TextMode=”MultiLine”
Width=”490px”></asp:TextBox></div>
</form>
</body>
</html>
STEP 4 – Write Below code in Msg.aspx.cs file at Page_Load Event
protected void Page_Load(object sender, EventArgs e)
{
string msg = (string)Application[“msg”];
txtmsg.Text = msg;
}
STEP 5 – Now, Design Chatting.aspx Page :
<body>
<form id=”form1″ runat=”server”>
<div>
<table style=”z-index: 100; left: 96px; position: absolute; top: 16px”>
<tr>
<td style=”height: 39px; text-align: center;” colspan=”2″>
<asp:Label ID=”lblwelcome” runat=”server” Font-Bold=”True” ForeColor=”Purple”></asp:Label><br />
<strong><span style=”font-size: 20pt; color: #0033cc”>Simple Chat Application in ASP.Net
C#</span></strong></td>
</tr>
<tr>
<td colspan=”2″ style=”height: 39px”>
<iframe frameborder=”no” height=”315″ scrolling=”no” src=”MSG.aspx” width=”515″>
</iframe>
</td>
</tr>
<tr>
<td colspan=”2″ style=”text-align: center”>
<table>
<tr>
<td style=”width: 100px”>
<asp:TextBox ID=”txtsend” runat=”server” Height=”50px”
TextMode=”MultiLine” Width=”384px”></asp:TextBox></td>
<td style=”width: 100px”>
<asp:Button ID=”btnsend” runat=”server” OnClick=”btnsend_Click” Text=”SEND” BackColor=”CornflowerBlue” Font-Bold=”True” Font-Size=”X-Large” ForeColor=”Black” Height=”56px” Width=”104px” /></td>
</tr>
</table>
</td>
</tr>
<tr>
<td style=”width: 100px”>
</td>
<td style=”width: 100px”>
</td>
</tr>
</table></div>
</form>
</body>
– The Out Put of Chatting .aspx is like :
STEP 6 – Write Below code at Page_load Event of Chatting.aspx Page.
protected void Page_Load(object sender, EventArgs e)
{
lblwelcome.Text = “Welcome ” + Session[“name”].ToString();
}
And the Last write Below code at BtnSend Msg Click Event of Chatting.asp.cs page.
protected void btnsend_Click(object sender, EventArgs e)
{
if (txtsend.Text != “”)
{
string name = Session[“name”].ToString();
string message = txtsend.Text;
txtsend.Text = “”;
string my = name + “::” + message;Application[“msg”] = Application[“msg”] + my + Environment.NewLine;
txtsend.Text = “”;
}
}
– After doing this things, you will enjoy online chat application in ASP.Net with C#.
very good codingg