ASP.Net – GridView Control
GridView control is used to display whole table data on web page. In GridView control each column define a filed or title, while each rows represents a record or data.
The GridView control dipaly data with rows and columns wise, we can display whole table in GridView and we also display only required columns from table in GridView control in asp.net.
In GridView control we can easily do sorting, paging and inline editing.
Also we can perform editing and deleting operation on displayed data with GridView control.
GridView Control Syntax :
<asp:GridView ID=”GridView1″ runat=”server”>
</asp:GridView>
GridView Example in ASP.Net C#
Now let’s take an asp.net example to bind data in to GridView.
Here, we learn how to bind and display data in GridView control from sql server database in asp.net c#.
GridView Control Example in ASP.Net C#
Step 1 – Open the Visual Studio –> Create a new empty Web application.
Step 2 – Create a New web page.
Step 3 – Drag and drop GridView Control on web page from toolbox.
Step 4 – Create New Database in SQL Server
Step 5 – Make connection between Database and web application.
In this asp.net example we will bind data to GridView from SQL server database. So, we need to do connection between our web application and sql server database. There are several method for make connection, we will explain three different connection method in our example.
- Bind data using SQL Connection and SQL DataAdapter
- Bind Data using DataSet and Table Adapter
- Bind Data using LINQ method.
ASP.Net GridView Control Example
Design asp.net web form with GridView control along with three button as show s in below figure.
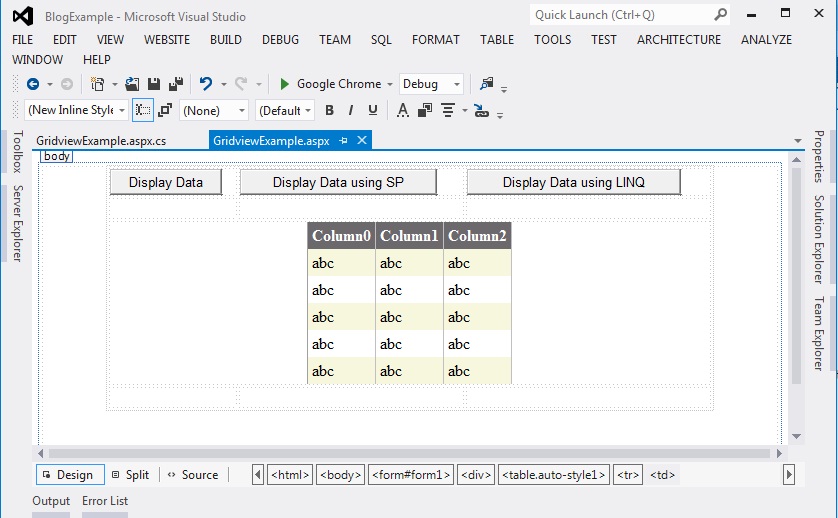
First add namespace on code page.
using System.Data.SqlClient;
using System.Data;
Bind data using SQL Connection and SQL DataAdapter
Now, write below code on ‘Display Data’ button.
This connection method we use SQLConnection and SQLDataAdapter object and write sql query in code behind page. This sql connection method are basic connection method.
protected void btnview_Click(object sender, EventArgs e)
{
SqlConnection SQLConn = new SqlConnection("Data Source=.\\SQLEXPRESS;Initial Catalog='Blog';Integrated Security=True");
SqlDataAdapter SQLAdapter = new SqlDataAdapter("Select * from UserMst", SQLConn);
DataTable DT = new DataTable();
SQLAdapter.Fill(DT);
GridView1.DataSource = DT;
GridView1.DataBind();
}
Bind Data using DataSet and Table Adapter
Write below code on ‘Display Data using SP’ Button.
In this method we use DataSet and SQL Stored Procedure for sql connection. Use Table Adapter instead of DataAdapter.
protected void btnviewdataSP_Click(object sender, EventArgs e)
{
DS_USER.USERMST_SELECTDataTable UDT = new DS_USER.USERMST_SELECTDataTable();
DS_USERTableAdapters.USERMST_SELECTTableAdapter UAdapter = new DS_USERTableAdapters.USERMST_SELECTTableAdapter();
UDT = UAdapter.SelectData();
GridView1.DataSource = UDT;
GridView1.DataBind();
}
Bind Data using LINQ method.
Write below code on ‘Display Data using LINQ’ button.
Here, we use LINQ method to fetch data from sql server and Display data in to GridView control.
protected void btnviewLINQ_Click(object sender, EventArgs e)
{
DataClassesDataContext Ctx = new DataClassesDataContext();
GridView1.DataSource = Ctx.USERMST_SELECT();
GridView1.DataBind();
}
Here is the result of above GridView example.
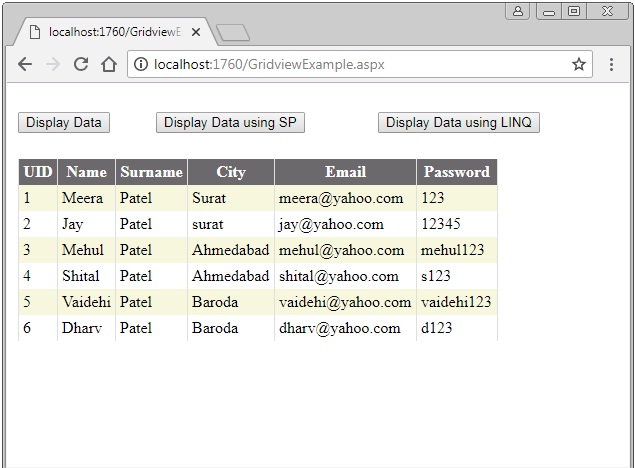
Full code of code behind page C# code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Data;
public partial class GridviewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnview_Click(object sender, EventArgs e)
{
SqlConnection SQLConn = new SqlConnection("Data Source=.\\SQLEXPRESS;Initial Catalog='Blog';Integrated Security=True");
SqlDataAdapter SQLAdapter = new SqlDataAdapter("Select * from UserMst", SQLConn);
DataTable DT = new DataTable();
SQLAdapter.Fill(DT);
GridView1.DataSource = DT;
GridView1.DataBind();
}
protected void btnviewdataSP_Click(object sender, EventArgs e)
{
DS_USER.USERMST_SELECTDataTable UDT = new DS_USER.USERMST_SELECTDataTable();
DS_USERTableAdapters.USERMST_SELECTTableAdapter UAdapter = new DS_USERTableAdapters.USERMST_SELECTTableAdapter();
UDT = UAdapter.SelectData();
GridView1.DataSource = UDT;
GridView1.DataBind();
}
protected void btnviewLINQ_Click(object sender, EventArgs e)
{
DataClassesDataContext Ctx = new DataClassesDataContext();
GridView1.DataSource = Ctx.USERMST_SELECT();
GridView1.DataBind();
}
}
Related ASP.Net Topics :
FileUpload Control
Button Control in ASP.Net C#
Subscribe us
If you liked this asp.net post, then please subscribe to our YouTube Channel for more asp.net video tutorials.
We hope that this asp.net tutorial helped you to understand about GridView control.
Next, asp.net tutorial we will understand about DetailView Control.