ASP.Net Application State
ASP.Net Application state is a server side state management technique.
Application state is a global storage mechanism that used to stored data on the server and shared for all users, means data stored in Application state is common for all user. Data from Application state can be accessible anywhere in the application. Application state is based on the System.Web.HttpApplicationState class.
The application state used same way as session state, but session state is specific for a single user session, where as application state common for all users of asp.net application.
Syntax of Application State
Store information in application state
- Application[“name”] = “Meera Academy”;
Retrieve information from application state
- string str = Application[“name”].ToString();
Example of Application State in ASP.Net
Generally we use application state for calculate how many times a given page has been visited by various clients.
Design web page in visual studio as shows in below figure.
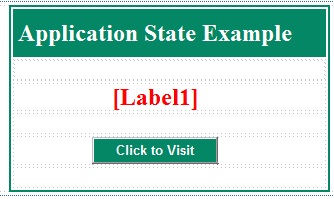
Here, we calculate total visit of uses visited web page by clicking “Click to Visit” button.
C# Code for Example
protected void btnvisit_Click(object sender, EventArgs e)
{
int count = 0;
if (Application["Visit"] != null)
{
count = Convert.ToInt32(Application["Visit"].ToString());
}
count = count + 1;
Application["Visit"] = count;
Label1.Text = "Total Visit = " + count.ToString();
}
Output of Example
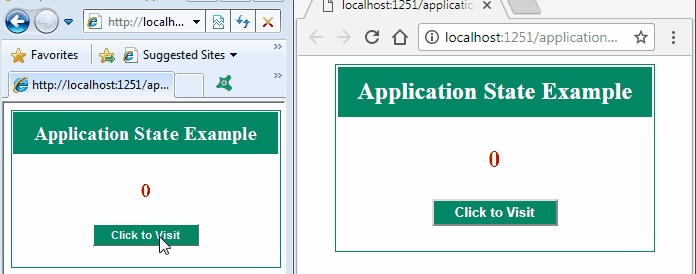
Here, above output screen we use different browser for visit same page. The result counter value stored in Application object so it would be changed simultaneously for both visitors.
In above case some time too many users click button at the same time, that time result wont be accurate. This situation known as dead lock. To avoid dead lock we use Lock() and UnLock() in application state.
Lock() and UnLock() in Application State
protected void btnvisit_Click(object sender, EventArgs e)
{
Application.Lock();
int cnt = 0;
if (Application["Visit"] != null)
{
cnt = Convert.ToInt32(Application["Visit"].ToString());
}
cnt = cnt + 1;
Application["Visit"] = cnt;
Application.UnLock();
Label1.Text = "Total Visit = " + cnt.ToString();
}
Session State Example in ASP.Net
Now, do above same example using Session state.
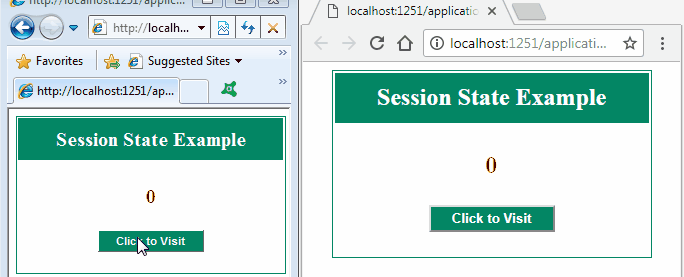
C# code for above example
protected void btnvisit_Click(object sender, EventArgs e)
{
int cnt = 0;
if (Session["Visit"] != null)
{
cnt = Convert.ToInt32(Session["Visit"].ToString());
}
cnt = cnt + 1;
Session["Visit"] = cnt;
Label1.Text = "Total Visit = " + cnt.ToString();
}
Related ASP.Net Topics :
Query String in C#.Net
Cookies in C#.Net
Subscribe us
If you liked this asp.net post, then please subscribe to our YouTube Channel for more asp.net video tutorials.
We hope that this asp.net c# tutorial helped you to understand about Application State in C#.
Next asp.net tutorial we will understand about ViewState in C#.