In this c#.net tutorial we will learn about double and float data types of variable with example.
Previous post we already learnt integer type variable and string type variable.
Float and double type variables
Float and double type variables are same as integer data types.
Integers are whole numbers. If we need to store numbers that are not whole numbers, we need to use double and float type of variable.
Float and double type of variable store point value something like : 8.10, 123.56.
Declaration of float and double type of variable are same as integer type of variable.
float floatvar;
double doublevar;
The difference between float and double is the size of the numbers that they can hold.
The float variable can have up to 7 digit numbers.
The double variable can have up to 16 digit numbers.
Float is a 32 bit number.
Double is a 64 bit number.
Example :
float fvar;
fvar = 18.5F;
The capital letter ‘F’ on the end means float variable. If you declare float without ‘F’ letter on end the c# compiler shows error message.
double dvar;
dvar = 18.5;
Here, double variable value no need to write ‘F’ at end of the value.
Now, let’s an asp.net c# example to more understand float and double variable.
ASP.Net C# Example
Open visual studio –> Create new website –> Add new web form –> Design form. Design web form with two button control one for get float value and second for get double value.
Here, we declare float and double variable with some values and get that variable value on web form while fire the button click event in c#.net like below screen.
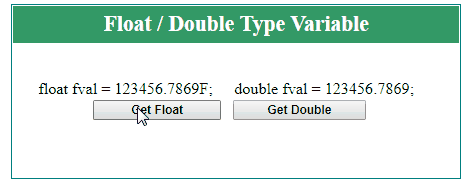
The c# code for above example:
protected void btnfloat_Click(object sender, EventArgs e)
{
float fval = 123456.7869F;
lblfloat.Text = "Float = "+ fval.ToString();
}
protected void btndouble_Click(object sender, EventArgs e)
{
double fval = 123456.7869;
lbldouble.Text ="Double = " + fval.ToString();
}
In above c# example we declare float variable fval = 123456.7869F. and display value in label control lblfloat when button btnfloat is clicked. Here we get result Float = 123456.8 because float variable can hold up to 7 digit numbers. The last 8th digit number is 8 so the 7 digit converted to 8 digit then the result is 123456.8.
Example :
float fval1 = 123456.7869F then the result = 123456.8
float fval2 = 123456.743F then the result = 123456.7
In double variable case we declare double variable dval = 123456.7869. same things done here as float variable and get result Double = 123456.7869. because double variable can have up to 16 digit numbers.
Related ASP.Net Topics :
Learn about Datatypes in C#
Learn about Variable in C#
Subscribe us
If you liked this asp.net post, then please subscribe to our YouTube Channel for more asp.net video tutorials.
We hope that this asp.net tutorial helped you to understand about float and double type variable in C#.
Next asp.net tutorial we will understand about Operator in C#.