In this asp.net c# post we will learn arithmetic operators with example.
Arithmetic Operators in C#.Net
As we know there are some basic arithmetic operations like Addition, Subtraction, Multiplication and Division are done by using arithmetic operators. Arithmetic operators are used for mathematical calculation in any programming. In this c#.net tutorial we will learn to use all the arithmetic operator with variable using c# language with example.
List of basic arithmetic operators are given below :
ID | OPERATOR | DETAIL | EXAMPLE |
---|---|---|---|
1 | + | Addition of Numbers | ans = number1 + number2 |
2 | – | Subtraction of Numbers | ans = number1 – number2 |
3 | * | Multiplication of Numbers | ans = number1 * number2 |
4 | / | Division of Numbers | ans = number1 / number2 |
5 | % | Reminder of Division | ans = 13%5 then ans=3 |
Addition operators (+)
The addition arithmetic operation done by “+” plus sign. Here, we add two variable value and store it in third answer variable and display result on screen.
Integer type variable addition
For doing addition arithmetic operation on variable first we declare variable then assign value to variable after we add up the variables. Here we declare two integer type variable firstno and secondno. we assign value to both variables 10 and 15 respectively as shows below.
int firstno;
int secondno;firstno = 10;
secondno = 15;
Now we want to add the firstno to secondno and the result will be stored in third variable we called addanswer.
The addition syntax is:
int addanswer;
addanswer = firstno + secondno;
addanswer = 10 + 15
addanswer = 25
Here we have a result of two variable addition is 25 as we use integer type variable.
The same example we done using string type variable the what happens lets see.
String type variable addition
Declare two string type variable with some values and add first no to second no and store result in third string variable.
string firstno;
string secondno;firstno = 10;
secondno = 15;
Here we use third string type variable to store the addition result.
string addanswer;
addanswer = firstno + secondno;
addanswer = 10 + 15
addanswer = 1015
Above code we get result in 1015 because the string type variable concatenate secondno to firstno.
Let’s implement integer and string type variables addition program in visual studio with c#.net.
Design web form as shows below screen along with two textbox control for input value and two button control one for integer addition and other for string addition. Bring two label control for display result one for integer result and other for string addition result.
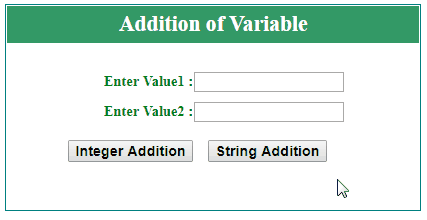
Above asp.net c# example as show screen we input two values 10 and 15 to textbox and on integer addition button we do integer type addition and on string addition button we do string type addition.
For integer addition 10 + 15 = 15
For string addition 10 + 15 = 1015
Here, is the c# code for above addition example
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnIntegeraddition_Click(object sender, EventArgs e)
{
int firstno;
int secondno;
firstno = Convert.ToInt32(TextBox1.Text);
secondno = Convert.ToInt32(TextBox2.Text);
int addanswer = firstno + secondno;
lblintegeranswer.Text ="Integer Sum = "+ addanswer.ToString();
}
protected void btnStringaddition_Click(object sender, EventArgs e)
{
string firstno;
string secondno;
firstno = TextBox1.Text;
secondno = TextBox2.Text;
lblstringanswer.Text ="String Sum = " + firstno + secondno;
}
Subtraction(-), Multiplication(*) Operators
Above example we learnt addition arithmetic operator, now here we do example for subtraction and multiplication operators.
Subtraction operator denoted by (-) minus sign.
Multiplication operator denoted by (*) star sign.
Subtraction and Multiplication C#.Net Example
Design asp.net web for same as above addition example for subtraction and multiplication.
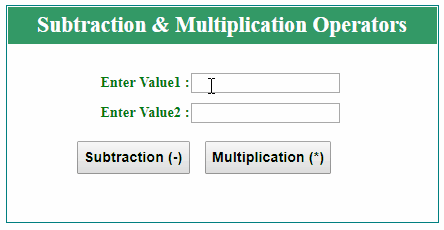
Above c# example we assign value 15 and 10 in textbox and we show the subtraction result 15-10 = 5 and multiplication result 15*10 = 150.
C# code for operators example
protected void btnsubtraction_Click(object sender, EventArgs e)
{
int firstno;
int secondno;
firstno = Convert.ToInt32(TextBox1.Text);
secondno = Convert.ToInt32(TextBox2.Text);
int answer = firstno - secondno;
lblsub.Text = "Subtraction = " + answer.ToString();
}
protected void btnmultiplication_Click(object sender, EventArgs e)
{
int firstno;
int secondno;
firstno = Convert.ToInt32(TextBox1.Text);
secondno = Convert.ToInt32(TextBox2.Text);
int answer = firstno * secondno;
lblmul.Text = "Multiplication = " + answer.ToString();
}
Division (/) and Module (%) Operators
Division operators denoted by (/) sign.
Module operators denoted by (%) sign.
Division and Module C#.Net Example
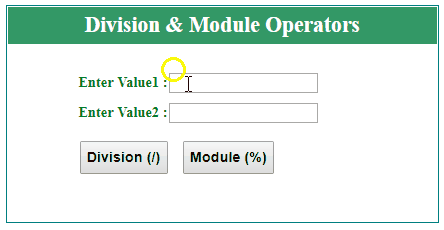
C# code for example
protected void btndivision_Click(object sender, EventArgs e)
{
int firstno;
int secondno;
firstno = Convert.ToInt32(TextBox1.Text);
secondno = Convert.ToInt32(TextBox2.Text);
int answer = firstno / secondno;
lbldiv.Text = "Division = " + answer.ToString();
}
protected void btnmodule_Click(object sender, EventArgs e)
{
int firstno;
int secondno;
firstno = Convert.ToInt32(TextBox1.Text);
secondno = Convert.ToInt32(TextBox2.Text);
int answer = firstno % secondno;
lblmod.Text = "Module = " + answer.ToString();
}
Related ASP.Net Topics :
Relational Operators in C#.Net
Assignment Operators in C#.Net
Subscribe us
If you liked this asp.net post, then please subscribe to our YouTube Channel for more asp.net video tutorials.
We hope that this asp.net tutorial helped you to understand about Arithmetic Operators in C#.
Next asp.net tutorial we will learn about Logical Operators.