C# Namespaces – Console Application
The namespaces helps to organize a programs. A namespace is designed for providing way to keep set of classes in a one organization. Namespace avoiding a clash of class by declaring same class name declared in another namespace.
Declaring Namespace :
A namespace definition starts with keyword namespace followed by the namespace name as like below:
namespace namespace_example
{
//programming code declaration
}
How namespace will helps:
For example in c# console application program, if we created same class name which is already available in .net framework class library, so there is a same class name clash problem occurs, but the namespace helps to avoid this kind of same declaration class name problem.
namespace MyFirstConsoleApp
{
class Program
{
static void Main(string[] args)
{
//code
}
}
}
In above example we defined namespace MyFirstConsoleApp with one class Program and a static function Main.
Here, we understand the same class name problem with an example.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace first_example
{
class first
{
public void firstfunction()
{
Console.WriteLine("The first namespacce example");
}
}
}
namespace Second_example
{
class first
{
public void firstfunction()
{
Console.WriteLine("The second namespacce example");
}
}
}
namespace MyExample
{
class Program
{
static void Main(string[] args)
{
first_example.first fst = new first_example.first();
fst.firstfunction();
Second_example.first sec = new Second_example.first();
sec.firstfunction();
Console.Read();
}
}
}
The output of above namespace example is:
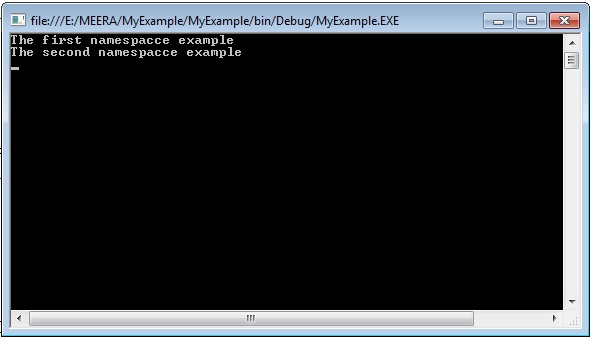
Nested Namespaces :
If we define one namespace inside the other namespace known as nested namespace.
Let’s take an example of nested namespace to understand more..
C# Nested Namespace Example
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace first_example
{
class first
{
public void firstfunction()
{
Console.WriteLine("The first namespacce example");
}
}
namespace Second_example
{
class first
{
public void firstfunction()
{
Console.WriteLine("The second namespacce example");
}
}
}
}
namespace MyExample
{
class Program
{
static void Main(string[] args)
{
first_example.first fst = new first_example.first();
fst.firstfunction();
first_example.Second_example.first sec = new first_example.Second_example.first();
sec.firstfunction();
Console.Read();
}
}
}
The output of above nested namespace example is:
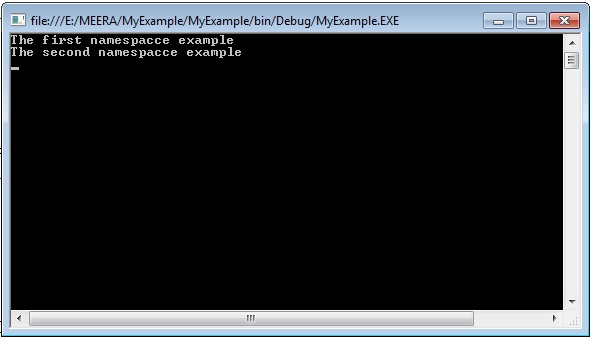
Related ASP.Net Topics :
Console Application in C#
Console Application Example
Subscribe us
If you liked this asp.net console application post, then please subscribe to our YouTube Channel for more asp.net video tutorials.
We hope that this asp.net post helped you to understand about Namespaces in Console Application.
Next, asp.net tutorial we will understand about Constructor in C#.