C# while loop
C# while loop used to execute same block of code again and again while a condition is true.
while loop syntax in C#
while(condition)
{
// block of code to be executed
}
In while loop first check the condition, if condition is true then execute a block of code.
Adding While loop in C# .Net
Open visual studio and create new website with c#. Open a web form code part and right click anywhere between brackets of the page load portion. On the right click menu select Insert Snippet and click on it as shows in below figure.
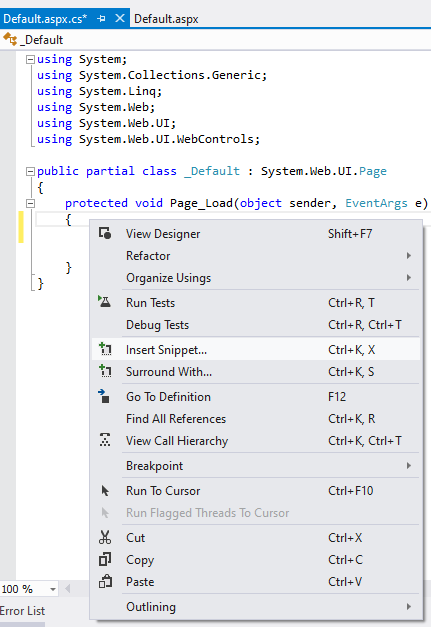
After click on Insert Snippet option from menu, you will see a list of items, Now double click on Visual C#.
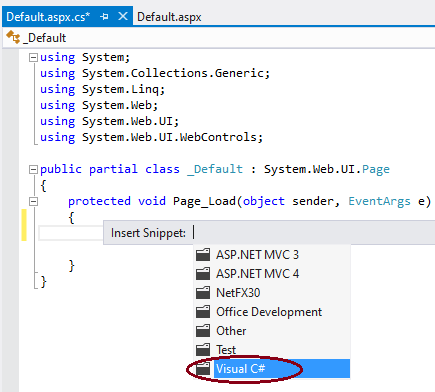
After double click on Visual C# option from items, you have list of snippets, Now double click on While.
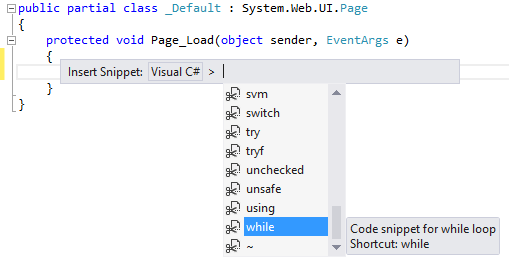
After double click on while option from list of snippets, you will have code of while loop on your web page.
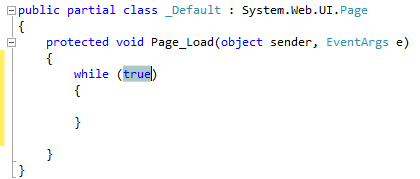
Example of C# while loop
int i=1;
while (i <= 5)
{
Response.Write(i);
i++;
}
The output is:
12345
In above for while example, first set integer variable i=1, the condition is i<=5,
for i=1 the condition is true then execute code print i.
Then increment 1 to i, ,
now i=2 the condition is true,
i=3 condition is true,
i=5 condition is true.
When i=6 the condition i<=5 is not true as 6 is not less than or equal to 5.
The while loop true for five times and the result is : 12345
C# while loop Example
int i=5;
while (i > 0)
{
Response.Write(i);
i--;
}
The output is:
54321
Here, we set integer variable i=5 and check condition i>0 after each time decrements 1 to variable i while a condition is true.
C# while loop Example
string name = "Meera Academy";
int i=1;
while (i <=5)
{
Response.Write(name + "<br/>");
i++;
}
The output is:
Hello Meera Academy
Hello Meera Academy
Hello Meera Academy
Hello Meera Academy
Hello Meera Academy
C# while loop example
int i=0;
int sum=0;
while (i <=5)
{
sum=sum+i;
i++;
}
Response.Write("The sum of 1 to 5 = " + sum);
The output The sum of 1 to 5 = 15
C# while loop Example
int i=1;
while (i <=10)
{
Response.Write(i);
i= i+2;
}
The output 13579
Example to display odd and even number using while loop.
string even="";
string odd="";
int i=1;
while (i <=20)
{
if(i%2==0)
{
even=even+" "+ i;
}
else
{
odd=odd+" "+ i;
}
i++;
}
Response.Write("The Even no = " + even +"<br />");
Response.Write("The Odd no = " + odd);
The output is:
The Even no = 2 4 6 8 10 12 14 16 18 20
The Odd no = 1 3 5 7 9 11 13 15 17 19
C# while loop example
int i=1;
while (i <=5)
{
int j = 1;
while(j<=i)
{
Response.Write(i);
j++;
}
Response.Write("<br />");
i++;
}
The output is:
1
22
333
4444
55555
Example
int i=1;
while (i <=5)
{
int j = 1;
while(j<=i)
{
Response.Write(j);
j++;
}
Response.Write("<br />");
i++;
}
The output is:
1
12
123
12345
PHP while loop example
int i=1;
while (i <=5)
{
int j = 5;
while(j>=i)
{
Response.Write(j);
j--;
}
Response.Write("<br />");
i++;
}
The output is:
54321
5432
543
54
5
Related ASP.Net Topics :
For Loop in C#.Net
Switch Statement in C#.Net
Subscribe us
If you liked this c#.net post, then please subscribe to our YouTube Channel for more c#.net video tutorials.
We hope that this asp.net c# tutorial helped you to understand about while loop.
Next asp.net tutorial we will learn about C# Do-While Loop.