In this asp.net c# post we will learn logical operators with example.
Logical Operators in C#.Net
The C# language supports basic three logical operators.The logical operators retursns true or false as output.
The logical operators are used when we have conditional statement such as if statement.
C# Supports following logical operators
Assume variable X is true and variable Y is false then
ID | OPERATOR | DETAIL | EXAMPLE |
---|---|---|---|
1 | && | Logical AND | if(X && Y) then false |
2 | || | Logical OR | if(X || Y) then true |
3 | ! | Logical NOT | if !(X && Y) then true |
Logical Operator &&
Logical operator && pronounced as AND operator. It returns true if both or all conditions are true and returns false is one of condition is false.
Assume int variable X=10 and variable Y=5 then
if ( X=10 && Y=4)
{
Result = “True”;
}
else
{
Result = “False”;
}
Here, output is Result = “False” because the second condition Y=4 is false.
Logical && operator example in visual studio with c# language.
Design web form like below screen along with two textbox, a button control and a label control. Textbox for username and password and label control for display result.
Here, we assume username=”meera” and password=”123″ when we try to login with same username and password we assumed then return message like welcome to system other wise return error message like invalid credential.
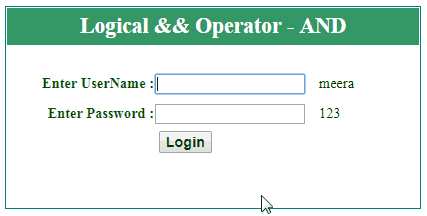
Here, we use && operator in if condition for check both username and password are right or wrong. Above c# .net example first we input username=meera and password=123 then result is Welcome to System and when we try username=meera and password=234 that time result will be Invalid Credential because password value is wrong as we assume.
C# code for above example :
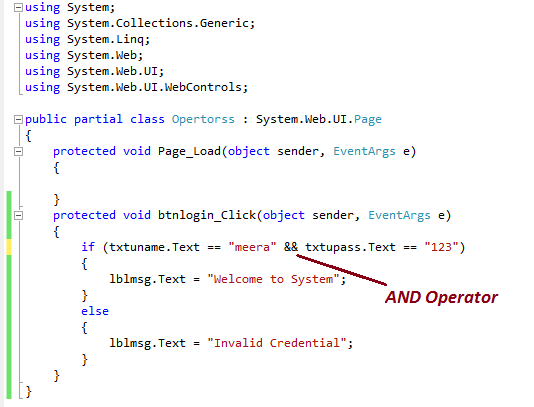
Logical Operator ||
The logical || operator pronounced as OR operator in c#.
As we learn above if we use && operator then both condition must be true but in || or operator one of single condition is true from all, it will display “Welcome to System” message.
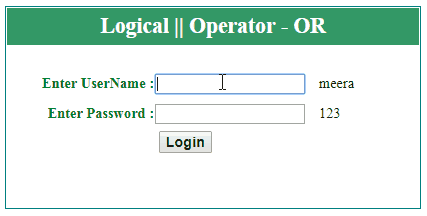
C# code for above example
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class Opertorss : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnlogin_Click(object sender, EventArgs e)
{
if (txtuname.Text == "meera" || txtupass.Text == "123")
{
lblmsg.Text = "Welcome to System";
}
else
{
lblmsg.Text = "Invalid Credential";
}
}
}
Logical Operator !
The logical operator ! pronounced as NOT operator in c#.
If the condition is true then logical not operator returns false.
Assume int variable X=10 and variable Y=5 then
if (X != Y)
{
result = “X is not equal to Y”;
}
else
{
result = “X is equal to Y”;
}
Here, output is result = “X is not equal to Y” because value of X and Y not same so return true block.
Related ASP.Net Topics :
Arithmetic Operators in C#.Net
Assignment Operators in C#.Net
Subscribe us
If you liked this asp.net post, then please subscribe to our YouTube Channel for more asp.net video tutorials.
We hope that this asp.net tutorial helped you to understand about Logical Operators in C#.
Next asp.net tutorial we will learn about Relational Operators.