Write a console application program in which accept two integer values and return sum, multiplication, division and subtraction of given values.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace MyExample1
{
class Program
{
static void Main(string[] args)
{
int a, b, sum, mult, sub;
float div;
Console.WriteLine("Enter Value of a and b\n");
a = Convert.ToInt32(Console.ReadLine());
b = Convert.ToInt32(Console.ReadLine());
sum = a + b;
Console.WriteLine("\nThe Sum of {0} and {1} = {2}\n", a, b, sum);
mult = a * b;
Console.WriteLine("The Multiplication of {0} and {1} = {2}\n", a, b, mult);
div = (float)a / b;
Console.WriteLine("The Division of {0} and {1} = {2}\n", a, b, div);
sub = a - b;
Console.WriteLine("The Substraction of {0} and {1} = {2}\n", a, b, sub);
Console.ReadLine();
}
}
}
The output of console application is :
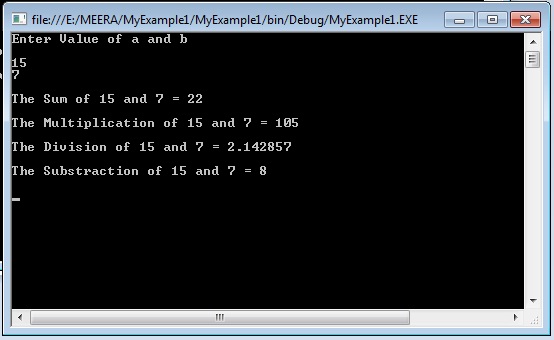
Related ASP.Net Topics :
Namespaces in Console Application
Constructor in Console Application
Subscribe us
If you liked this asp.net console application post, then please subscribe to our YouTube Channel for more asp.net video tutorials.
We hope that this asp.net post helped you to understand about console application example in C#.
Next, asp.net tutorial we will understand about Visual Studio.