In this asp.net c# post we will learn assignment operators with example.
Assignment Operators in C#.Net
C# Supports following Assignment operators
ID | OPERATOR | DETAIL | EXAMPLE |
---|---|---|---|
1 | = | value assign from right to left operand | (X = Y) |
2 | += | Add and assign | (X += Y) means X = X + Y |
3 | -= | Subtract and assign | (X -= Y) means X = X – Y |
4 | *= | Multiply and assign | (X *= Y) means X = X * Y |
5 | /= | Divide and assign | (X /= Y) means X = X / Y |
6 | %= | Modulus and assign | (X %= Y) means X = X % Y |
Let’s c# example to more understand about assignment operators.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class Default4 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnresult_Click(object sender, EventArgs e)
{
int a = Convert.ToInt32(txtno1.Text);
int b = Convert.ToInt32(txtno2.Text);
a = b;
lbl1.Text = "Assign a = b :: " + a.ToString();
a += b;
lbl2.Text = "Add and assign a += b :: " + a.ToString();
a = Convert.ToInt32(txtno1.Text);
a -= b;
lbl3.Text = "Subtract and assign a -= b :: " + a.ToString();
a = Convert.ToInt32(txtno1.Text);
a *= b;
lbl4.Text = "Multiply and assign a *= b :: " + a.ToString();
a = Convert.ToInt32(txtno1.Text);
a /= b;
lbl5.Text = "Divide and assign a /= b :: " + a.ToString();
a = Convert.ToInt32(txtno1.Text);
a %= b;
lbl6.Text = "Modulus and assign a %= b :: " + a.ToString();
a = Convert.ToInt32(txtno1.Text);
}
}
The output of c# program is :
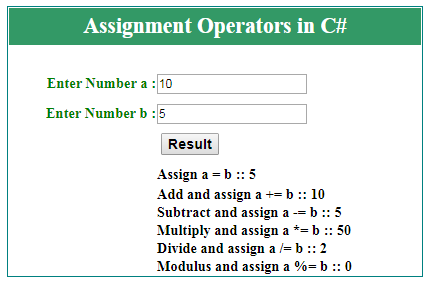
Related ASP.Net Topics :
Arithmetic Operators in C#.Net
Logical Operators in C#.Net
Subscribe us
If you liked this asp.net post, then please subscribe to our YouTube Channel for more asp.net video tutorials.
We hope that this asp.net tutorial helped you to understand about Assignment Operators in C#.
Next asp.net tutorial we will learn about C# Conditional Statement.